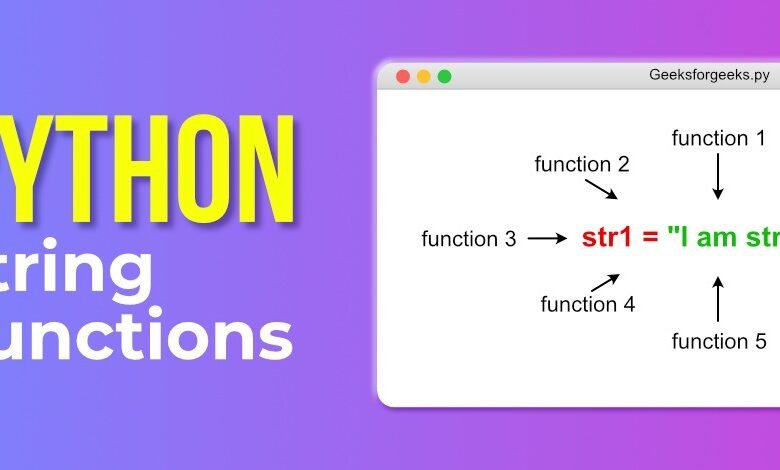
String functions are tools available in programming and computing that manipulate, analyze, or modify text (strings). The functionality and syntax can vary depending, but the core concepts are often similar across different programming languages. Here’s an overview of commonly used string functions and their purposes:
1. Length
This function returns the number of characters in a string. It’s used to determine the length of the text data.
- Example in Python:
len("Hello")
2. Concatenation
Concatenation combines two or more strings into one. This is used to join text data.
- Example in Python:
"Hello" + " " + "World"
3. Substring
This function extracts a portion of a string, typically by specifying starting and ending positions or just the start position and length.
- Example in Python:
"Hello World".substring(0, 5) # Results in "Hello"
4. Replace
Replace is used to substitute all or part of a string with another string. It’s useful for modifying text.
- Example in Python:
"Hello World".replace("World", "Everyone")
5. Split
Split divides a string into a list based on a delimiter (a specified character or characters used to define the split points).
- Example in Python:
"apple, banana, cherry".split(", ")
6. Trim (or Strip)
This function removes whitespace or specified characters from the beginning and end of a string.
- Example in Python:
" Hello World ".strip()
7. ToUpper and ToLower (Case Functions)
These functions change the case of the letters in a string, converting all to uppercase or lowercase, respectively.
- Example in Python:
"Hello World".upper() # HELLO WORLD
"Hello World".lower() # hello world
8. IndexOf and LastIndexOf
These functions return the position of a specified character or substring within a string. If the character or substring isn’t found, they typically return -1.
- Example in Python:
"Hello World".index("o") # Returns 4
"Hello World".rindex("o") # Returns 7
9. StartsWith and EndsWith
These functions check if a string starts or ends with a specified substring.
- Example in Python:
"Hello World".startswith("Hello") # True
"Hello World".endswith("World") # True
10. Format
This function is used to insert values into a string placeholder, useful for creating strings that include variable data in a template.
- Example in Python:“The quick {0} fox jumps over the {1} dog”.format(“brown”, “lazy”)
Application in Different Contexts
String functions are integral in data processing, web development, automation scripting, and more. They enable programmers to handle text efficiently, whether it’s parsing user inputs, generating text outputs, manipulating data retrieved from databases, or customizing messages shown to users.
Each programming language might have specific variations or additional string functions tailored to common tasks within that language’s ecosystem. Always check the specific documentation for details on how to use these functions effectively in your chosen language.